views
Streamlining Your Flask Application with Playwright and Pytest: A Step-by-Step Guide to Automation Testing
In today's fast-paced software development world, automation testing plays a pivotal role in ensuring high-quality applications. Flask, a popular micro web framework for Python, has become a go-to choice for developers seeking simplicity and flexibility in their web applications. However, as your application grows, testing becomes an essential part of your workflow, and that’s where Flask Pytest comes in.
This article will guide you through the process of automating testing for your Flask application using Playwright and Pytest. We'll explore practical examples to help you seamlessly integrate these tools into your testing framework. By the end, you'll have a clear understanding of how to harness the power of these technologies to streamline your testing process and improve the reliability of your Flask applications.
What Is Flask Pytest?
Flask Pytest refers to the combination of Flask (a micro-framework for Python) and Pytest (a popular testing framework). Pytest is widely known for its simplicity and rich set of features, which makes it a great fit for testing Flask applications. Whether you're testing APIs or rendering templates, Flask Pytest enables you to write concise, readable tests with minimal effort.
Why Use Playwright for Automated Testing?
Playwright is a powerful browser automation tool that allows you to script interactions with web applications across multiple browsers (Chromium, Firefox, and WebKit). Playwright enables you to simulate real-world user behavior, like clicking buttons, filling out forms, and navigating pages, all within your test scripts.
When combined with Flask and Pytest, Playwright can be an invaluable tool for automating end-to-end testing of your web applications. It ensures your application behaves as expected across all browsers and can help catch potential issues before they reach production.
Setting Up Your Flask Application for Testing
Before diving into the specifics of Flask Pytest and Playwright, it’s important to set up a basic Flask application. Let’s start by creating a simple Flask app that we can later test.
-
Install Flask: Begin by installing Flask using pip:
pip install Flask
-
Create Your Flask App: Create a simple Flask app in a Python file (e.g.,
app.py
):from flask import Flask app = Flask(__name__) @app.route('/') def home(): return "Hello, Flask!" if __name__ == "__main__": app.run(debug=True)
-
Run the Flask App: Run the Flask application locally by executing the following command:
python app.py
Now that your Flask application is set up, you can move on to setting up testing with Playwright and Pytest.
Automating Tests with Pytest
Pytest simplifies the process of writing tests for Flask applications. To begin, you’ll need to install Pytest:
pip install pytest
Writing Tests for Flask with Pytest
Here’s how you can write basic tests for your Flask application using Pytest. Start by creating a new file called test_app.py
and add the following:
import pytest
from app import app
@pytest.fixture
def client():
with app.test_client() as client:
yield client
def test_home(client):
response = client.get('/')
assert response.data == b"Hello, Flask!"
assert response.status_code == 200
In this test, we’re verifying that the root URL (/
) returns the correct message and status code.
Adding Playwright for End-to-End Testing
Next, we’ll integrate Playwright into our testing setup to perform more comprehensive end-to-end tests. Begin by installing Playwright:
pip install playwright
playwright install
Using Playwright with Pytest
To use Playwright with Pytest, you can set up a simple Playwright test that interacts with your Flask application. Here's an example of using Playwright to test the homepage:
import pytest
from playwright.sync_api import sync_playwright
def test_homepage_with_playwright():
with sync_playwright() as p:
browser = p.chromium.launch()
page = browser.new_page()
page.goto("http://127.0.0.1:5000")
assert page.content() == "<html><body><h1>Hello, Flask!</h1></body></html>"
browser.close()
This test opens your Flask application in a Chromium browser and verifies that the content of the page matches the expected HTML.
Integrating Testomat for Advanced Test Management
When managing your tests and test cases, Testomat.io comes into play as an essential tool. Testomat allows you to automate the management and execution of your tests, providing a centralized platform for tracking results and organizing your test cases.
Why Testomat.io?
-
Centralized Test Management: Organize and track your automated tests in one place.
-
Real-Time Insights: View the status of your test cases and the performance of your applications.
-
Efficient Collaboration: Share test reports and collaborate with your team seamlessly.
In addition to Testomat.io, here are some other tools that can aid in your testing process:
-
Testomat.io: Comprehensive test management platform.
-
Playwright: Browser automation tool for end-to-end testing.
-
Pytest: Testing framework for Python applications.
-
Flask: Python micro web framework.
-
Selenium: Another popular browser automation tool.
Running Your Tests and Managing Results
Once your tests are written and automated, you can execute them using Pytest by running the following command:
pytest
You will see output detailing which tests passed and which failed. For a more comprehensive testing workflow, Testomat.io offers easy integration with Pytest and Playwright, giving you a clear overview of your testing progress and results.
Best Practices for Flask Pytest Automation
While automating your tests with Flask and Pytest is essential, it’s also crucial to follow best practices to ensure your tests are efficient and maintainable:
-
Test Coverage: Ensure you’re testing all critical paths in your Flask application, including APIs, templates, and forms.
-
Keep Tests Small and Focused: Write small, focused tests that check one piece of functionality at a time.
-
Use Fixtures for Setup: Leverage Pytest fixtures for test setup, ensuring your tests are isolated and easy to maintain.
-
Test Locally Before Deploying: Always run your tests locally before deploying them to production to catch potential issues early.
Conclusion
Automating tests for your Flask application using Pytest and Playwright will significantly enhance the reliability and quality of your codebase. With the help of tools like Testomat.io, managing and tracking your tests becomes seamless, ensuring that you can maintain high-quality standards across your application development lifecycle.
For more details, you can explore the full guide on Flask Pytest automation testing to gain further insights into automating your Flask application testing.
By incorporating these tools and practices into your development process, you'll not only improve the efficiency of your testing but also ensure a smooth and error-free user experience.

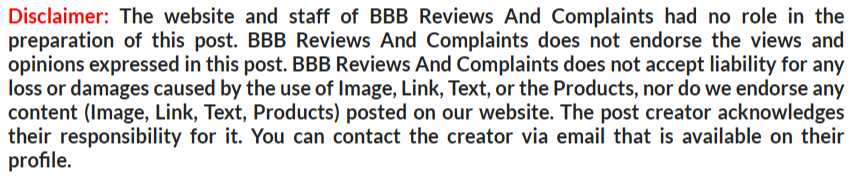
Comments
0 comment